Log
View Options
UTF32 to UTF16 Surrogate Pairs
10/11/22
Recently I had to make an Icon component that could accept UTF32 characters to display in SVG glyphs. It seemed pretty simple at first. I already had an array of unicode symbols I would plug in and my component would represent them in an ADA friendly way.
Then I noticed the list contained several character codes that were out of the UTF16 scope and just as I feared these got garbled when trying to run them through JavaScript. JavaScript being built long before emojis and the need of UTF32 only supports UTF16 in strings to this day (sort of).
Eventually when UTF32 started becoming more popular the Unicode maintainers came up with a solution. There where several plains or ranges of higher character codes that weren't currently in use. While these could in no way to support a one to one mapping to accommodate UTF32 they decided they would make supplementary character sets using two 16 byte sets. After some searching I finally found a table of surrogate characters.
Now, I figured surely, there is a built in solution in the modern era for supporting UTF32 in JavaScript and there is. The native String object has a method called fromCodePoint which is supported by all major modern browsers.
Sure enough, I was able to plug my UTF32 characters and return the two UTF16 characters that would make my glyphs. Cool, but, it left me curious. The only way to use UTF32 characters in JavaScript before was to use fromCharCode. So, how did the math work to know how to split the UTF32 values when using fromCharCode?
After much digging I found an example of the alogrithm on the Unicdoe Consortium website. The only issue was it was not very well documented as to why certain operations were being performed. I needed to know more.
After much searching, trial, and error I came across a Wikipedia article that helped me understand just why all of this math was necessary and how it worked to reorder the bytes. I ended up developing a well commented example function for converting UTF32 to UTF16 friendly characters. While this function seems to work well I would recommend the Mozilla polyfill if you're to need something like this for production.
[JavaScript]
/* @summary: Take a unicode character code. If it is 16bit range do nothing. If it's in 32 bit range convert to a UTF32 JavaScript friendly character set.
* @prop c: A character or a number to covert to a JavaScript friendly character.
*/
function asUTF16(c)
{
// Minimum value for the high end character code (fist 10 digits)
const surh_base = 0xd800;
// Minimum value for the low end charater code (last 10 digits)
const surl_base = 0xdc00;
// Row size for surrogate characters
const sur_int = 0x400;
// The leading bit to remove before math operations
const xbit = 0x10000;
// The stripped down value for calcualting surrogate
let c_base = (c - xbit);
// First value determined by left 10 bits plus surrogate high base
let c1 = Math.floor(c_base / sur_int) + surh_base;
// If c1 is not a 32bit range return the original 16bit set
if(c1 < surh_base)
return String.fromCharCode(c);
// Second value determned by right 10 bits plus surrogate low base
let c2 = (c_base % sur_int) + surl_base;
// Return our 32bit character set
return String.fromCharCode(c1, c2);
}
Try it out
Table Top Map Maker
8/25/20
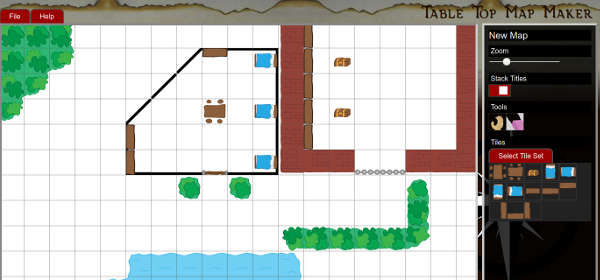
A while back I started keeping a web page to track of my D&D games. It made running games a lot easier to have all of my information in one place. The only real downside was making maps. For a while I would just carry my custom maps in a folder. Then, wanting to have all of my info in one place, I started taking pictures of the maps and uploading them to my server. While it worked, it was not an optimal solution.
After a while I though it would be more convenient to have an application to make maps, save them for editing, and export JPG files. Well, I started out on this a little over a month ago (working in my spare time) and have come up with the beginning of something that has a lot of potential.
Currently, the interface is simple enough. On the right side is a select menu with different tile sets you can pick from. Click on your tile set and then click where you want to set it. There are some simple features like zoom (in and out), a toggle for stacking tiles, a grabber and an eraser.
There is basic file management functionality that lets you create a new map, save a map for editing, load a saved map, and export a map to a JPG. The save files use a JSON format to store the SVG data and repopulate it on load.
One feature that I'm excited about is how the master SVG tiles get loaded into the application. In the ./tiles directory there is a file name tile_sets.json that allows anyone to include more tiles, thus allowing creates to make and share their own tile sets!
When making a tile set you can start with the mm_tiles_templates.svg (or .ai) make a copy and name it. Then you'll see several layers (<g> objects) each with an id that signifies where the tiles relative placement is. There is also a guides layer that will not render into a tile in the application. This is just to help tile authors line up their edges.
The application is in a usable state currently and you can make, save, and export maps. The next steps are making the design look better; I sort of slopped it together as I developed. Then I'm going to work on making it mobile friendly. After all of that, I plan of nailing down the Help instruction and committing it to my new Cyber Monkey GIT share so people get build new tiles and features.
Anyways, if you are interested making some maps or just want to play with the app, check it out here!
Dynamic SVG Filters
6/3/20
When redesigning my (this) site recently I wanted to do some fun things with dynamic display using SVG elements. If you have noticed the background is always different shapes and colors. This is because it is made up of randomly generated SVG elements. You may also notice that there is a shine to some of the edges. This is because of dynamically generated SVG filters applied to the shapes.
Here I'm going to share the techniques on making your own dynamic SVG filters. You will need some understanding of SVG filters to follow along. For this exercise we're going to create a light filter like the example below.
Light Filter Controls
Step 1: Element Setup
Let's set up our elements. First we'll add a placeholder element where we'll add our SVG content later.
[HTML]
<div id="svg_wrap">
<!-- Dynamic content -->
</div>
It's important to note SVG uses
DOM2
which means referencing them in JavaScript isn't exactly the same as referencing a HTMLElement.
To create an SVG element we'll want to specify the namespace where our element is defined. For all SVG elements you can use http://www.w3.org/2000/svg. To apply the namespace when creating elements we'll use createElementNS. Let's start and build all of the basic SVG elements.
- <svg> The main SVG element that will hold all other elements.
- <defs> Here we define filters that we can attach to our graphic elements. Child of svg.
- <filter> The filter combines all of the style layers that we will want to apply. Child of defs.
- <feSpecularLighting> A basic lighting layer that controls color and intensity. Child of filter.
- <fePointLight> A modifier for feSpecularLighting that controls size and placement. Child of feSpecularLighting.
- <feComposite> This allows us to add a filter to a graphic object. Child of filter.
- <circle> This is our graphic element. Child of svg.
Let's see what this looks like in script.
[JavaScript]
var svg = document.createElementNS("http://www.w3.org/2000/svg", "svg");
var defs = document.createElementNS("http://www.w3.org/2000/svg", "defs");
var filter = document.createElementNS("http://www.w3.org/2000/svg", "filter");
var fe_spec_light = document.createElementNS("http://www.w3.org/2000/svg", "feSpecularLighting");
var fe_point_light = document.createElementNS("http://www.w3.org/2000/svg", "fePointLight");
var fe_comp = document.createElementNS("http://www.w3.org/2000/svg", "feComposite");
var circle = document.createElementNS("http://www.w3.org/2000/svg", "circle");
Cool, now we have all of the elements we'll be using to build our example.
Step 2: Attribute Setup
Next we'll set the attributes to our elements. Unlike the SVG elements, a namespace isn't required when manipulating element attributes. So, when calling setAttributeNS the first parameter will be null.
I have added comments to the code sample below to explain some of the more esoteric attributes.
[JavaScript]
// Set attributes for the svg element
svg.setAttributeNS(null, "viewBox", "0 0 100 100"); // The display area of our SVG canvas
svg.setAttributeNS(null, "width", "100"); // The width of our SVG canvas
svg.setAttributeNS(null, "height", "100"); // The height of our SVG canvas
// Set attributes for the filter element
filter.setAttributeNS(null, "id", "circle_fitler"); // For refercning when applying the filter to our circle element
// Set attributes for the feSpecularLighting element
fe_spec_light.setAttributeNS(null, "result", "spec_light"); // For refercning in feComposite in2 attribute
fe_spec_light.setAttributeNS(null, "specularConstant", "2"); // The hardness of the light
fe_spec_light.setAttributeNS(null, "specularExponent", "50"); // The spread of the light
fe_spec_light.setAttributeNS(null, "lighting-color", "#fff"); // The color of the light
// Set attributes for the fePointLight element
fe_point_light.setAttributeNS(null, "x", "50"); // The X position of the light
fe_point_light.setAttributeNS(null, "y", "20"); // The y position of the light
fe_point_light.setAttributeNS(null, "z", "50"); // The z or distance of the light
// Set attributes for the feComposite element
fe_comp.setAttributeNS(null, "in", "SourceGraphic"); // The element to apply the filter to. SourceGraphic means the origin element
fe_comp.setAttributeNS(null, "in2", "spec_light"); // The id of the filter to apply
fe_comp.setAttributeNS(null, "operator", "arithmetic"); // This allows us to combine the outcomes of our two in targets
fe_comp.setAttributeNS(null, "k1", "1"); // Light source inside of SourceGraphic
fe_comp.setAttributeNS(null, "k2", "1"); // SourceGraphic opacity
fe_comp.setAttributeNS(null, "k3", "0"); // Setting this to 0 prevents the transparent background from getting light
fe_comp.setAttributeNS(null, "k4", "0"); // Setting this to 0 prevents the transparent foreground from getting light
// Set attributes for the circle element
circle.setAttributeNS(null, "cx", "50"); // Start X position
circle.setAttributeNS(null, "cy", "50"); // Start Y position
circle.setAttributeNS(null, "fill", "rgb(153, 153, 255)"); // Circle fil color
circle.setAttributeNS(null, "r", "50"); // Circle radius
circle.setAttributeNS(null, "style", "filter: url(#circle_fitler);"); // Point to our filter id to apply it
Step 3: Construct the Elements
Now we just need to put the elements together in the right order. Use the elements list above if you get confused on what element should be a child of another element.
[JavaScript]
fe_spec_light.appendChild(fe_point_light);
filter.appendChild(fe_spec_light);
filter.appendChild(fe_comp);
defs.appendChild(filter);
svg.appendChild(defs);
svg.appendChild(circle);
// Target the element where we add all of the elemtns
let svg_wrap = document.getElementById("svg_wrap"); // Use the id of the element you want to add everything to
svg_wrap.appendChild(svg);
Now you should be able to run your code and see your circle with the pin light filter applied to and since we have JavaScript references to all of the elements we just built we can easily change their attributes.
Step 4: Applying Controls
Now, there are a whole bunch of ways we could manipulate our light. We could use a setTimeout to gradually move it. We could make it blink. We could make it pulse. For the purposes of this experiment we're going to use range sliders to adjust our light.
Here is the markup for the range sliders.
[HTML]
<div>
<input id="pinx" name="pinx" type="range" min="0" max="100" step="1" value="50"/>
<label for="pinx">X</label>
<br/>
<input id="piny" name="piny" type="range" min="0" max="100" step="1" value="20"/>
<label for="piny">Y</label>
<br/>
<input id="pinz" name="pinz" type="range" min="0" max="100" step="1" value="50"/>
<label for="pinz">Radius</label>
</div>
Note the id attribute of the inputs. We'll be using these.
[JavaScript]
// Get referces to control inputs
ctrl_pinx = document.getElementById("pinx");
ctrl_piny = document.getElementById("piny");
ctrl_pinz = document.getElementById("pinz");
// Create contorl function
function updateFitlers(e)
{
let fx = ctrl_pinx.value;
let fy = ctrl_piny.value;
let fz = ctrl_pinz.value;
fe_point_light.setAttributeNS(null, "x", fx + "");
fe_point_light.setAttributeNS(null, "y", fy + "");
fe_point_light.setAttributeNS(null, "z", fz + "");
};
// Assign functionality
ctrl_pinx.addEventListener("input", updateFitlers);
ctrl_piny.addEventListener("input", updateFitlers);
ctrl_pinz.addEventListener("input", updateFitlers);
Conclusion
Congratulations, if you've been following along you should have your own dynamic SVG filter working. If not you can download the example package and have some fun with it.
Action JavaScript
11/2/19
Now that ECMA 6 JavaScript standards are widely supported on most browsers I wanted to start a project that would help me explore the intricacies of the new capabilities. Being an old ActionScript expert and fan of game development I thought developing an AS3 like set of libraries would be a fun an interesting project. Currently, for lack of a better name I'm calling it ActionJavaScript ... at least until I find a better title. The project is currently running under GNU GPLv3 so, ya know, do as you will with it.
I currently have a good enough foundation to create web games with relative ease. My test game for the project is Mad Monkey and the Thunderdome which I plan on building up along with the AJS libraries.
Here is a list of the current libraries available.
Currently manages basic events and object hitTests.
Stage - scripts/ui/stage.js
Just as in Flash the Stage object is the main container for all other UI element and is essentially a glorified MovieClip.
MovieClip - scripts/ui/movieClip.js
An object with a set of frames and frame rate that and play or be controlled via script. The use of "ENTER_FRAME" events allows for easy control.
Frame - scripts/ui/movieClip.js
The hold the different "frames" used in movie clips and can consist of a group of text or images.
BitMap - scripts/ui/bitMap.js
A standard pixel based image similar to a JS Image object.
TextField - scripts/ui/textField.js
Used for adding and controlling text object.
Button - scripts/ui/button.js
An interactive UI component for click events. Buttons currently have four states that can be styled [up, over, focus, active].
So far creating my little test game took less than a day and one I get this project moved forward a little more I would like to start making move browser based games using it. I think the time is long overdue for a Skate-er-Guy 2.
If all of this sounds like something you'd like to play with you can download AJS along with my test game HERE.
CSS/JS 3D Cube
9/2/16
Check out these examples.
Introducing PolySure
5/13/15
After continually being frustrated over lack of cross-browser support of loads of basic JavaScript functions I got into extending prototypes and more importantly, polyfills. I made some simple ones, but then found Mozilla Developer Network provided some very useful ones like Function.bind and Object.create. I have been putting together a self executing JavaScript function that adds several polyfills if the functionality doesn't exist. The name of the function? PolySure.
In all seriousness though, PolySure can help your web development experience by providing cross-browser and backward compatible JavaScript. As of writing this the current polyfills supported are:
- console.log
- Array.indexOf
- Array.lastIndexOf
- Object.create
- Function.bind
- Array.isArray
- all JSON functions/objects
Feel free to download PolySure for yourself (at your own risk).
Custom Form Check Boxes
6/25/13
I found my self wanting to style form check boxes, however after looking around a bit on-line I didn't come across any simple CSS solutions. Of the solutions I did find, many of them were Web-Kit reliant and didn't work very well in older browsers. I made this simple JS class to allow custom styling of check boxes.
The class it's self is pretty straight forward. You give it a name(id), title(label), up HTML, selected HTML, over HTML, and CSS class and it returns a custom check box object. After your check box is created you can then append it to whatever form you need via the JavaScript appendChild() command. It is also a good idea, if you are using images, to preload any images you may want to use (see example package).Select fruits
Here is a basic example of using the class.
Update
I ended up finding a pretty nice CSS based checbox styling tutorial.
HTML Color Selector
6/17/13
The other day I was playing around with my old canvas drawing class and thought to myself, I really could add more colors to this thing. Quickly using some loops to generate a number of color tabs I realized with only a little more effort I could make a full-on color selector class. So I did.
The algorithm isn't perfect but it does contain an extensive array of colors. You can pass two parameters to the class when instantiating. The fist (threshold) determines how many steps in color value the color selector will take between drawing color tiles. For example a threshold of 8, getting lighter, would build color tiles like so ((239,156,16), (247,164,24), (255,172,32)). The second parameter sets the square pixel size of the color tiles. If you don't set these they default to 8 and 2 respectively.
Here is an example of using this class...
... and this is how it's built on this very page.
In the second code snippet you see I'm doing a couple of things with the class before I call the init method. There are a number of public methods and event listeners you can use to interface with the CMDColorSelector.
* init : build the color selector to the document/parent
* select_color : returns the last color clicked on by user
* active_color : returns the value of the color the mouse last hovered over
* threshold : returns the threshold step value. threshold must be set on instantiation
* tab_size : retuns the sqare pixel size of the color tabs. tab_size must be set on instantiation
* inititalized : boolean, true if init() has been called
* parent : allows you to set the parent element the CMDColorSelector will be built on.
also can be used to get the current parent if no parameter is passed in.
you cannot set the parent if initialized is true
* RGBtoHEX : converst an rgb(0,0,0) based string to a hexidecimal based string
* setEvent : allows for stored functions to be called during certain class events.
The first param is the event type and the second is the function to be called (see EVENTS)
EVENTS
* onInit : executes at the end of the init() method
* onUpdateSC : executes whenever the selected_color value changes
* onUpdateAC : executes whenever the active_color value changes
Along the way I leaned two interesting things. First, IE (save for v8) has no support for the standard getter and setter methods that other browsers use, and the amount of code it takes to fix that issue just does not seem worth the effort and processing power. Second, in most versions of IE and Safari, mouse event bubbling (or registering) might not happen properly for child elements when their parent has a CSS display value of "block". To get around the second issue I set the parent elements CSS pointer-events value to none, while setting all of the clickable child elements pointer-events value to all.
In the (hopefully near) future I will be adding this color selector to the canvas drawing class. Who knows, with a couple more features I could have an on-line version of MS-Paint!
JavaScript Accordion
5/7/13
As a developer I have to often take "coding challenges". A recent one had a static picture of a menu that looked like an accordion menu. Being a coding challenge I didn't want to use some giant jQuery package, nor did I want to build a function to toggle each menu. The solution was obviously my own custom JavaScript class that modified the Document Object Model (DOM) based on simple naming conventions.
The JavaScript class will scan the DOM for any div tags with a class attribute value of "accordion_menu". Once an accordion_menu div is identified the AccordionManger class will turn every odd child div into an clickable title that will toggle the display of the proceeding even div tag.
Here is the source that made the above menu.
I have tested this in Opera, Firefox, IE, and Chrome. It seems to work fine in all of them, even older versions. However use at your own risk, it is just an example after all. If you would like to use or expand on this little class, download the example package.
Dynamic Draggable Divs
7/12/11
Since I often need to create dynamic javascript created divs, I decided to make a class that is easily adapatable. While I was at it I decided to add some simple features like mouse dragging and easy data editing. Things are still in the works with this one, however use the text area below to generate a floating div with content. Then take your mouse and drag it around a bit.
There are still some bugs to work out for Internet Explorer. When I get a semi-stable version I'll try and post up some source code and examples.
HTML5 Canvas Drawing
5/20/11

It's an Indian, it's a wolf? It was made with my latest Javascript class. I have been doing more experiments with the HTML5 canvas object. This time I have made a pretty fun drawing class. It's very basic and only offers the ability to change color, size, and brush. However a start it is!
There is still much tweeking needed to make drawing smooth. Look for updates like an actual outline as to your brush area and better brush spread. Right now drawing is based off of the mouseMove javascript event. I plan on changing this to a rapid setInterval event that should allow for a nicer flow of the brush head.
This does not work in IE and you may experiance problems in Safari, so you might want to try it in
AnimatedImg - Javascript Class
4/20/11
Some time ago I had an urge to create an animated set of .png images. Well, in the fun of experimentation I went a little over board and made a full javascript class that not only animates several different types of image files, but also offers a nice set of play control methods and event listeners!
Ok, lets go over a little of how it works, shall we? First lets create a simple animation of a ball bouncing using. There are only three basics things we need here. One, an array of image files. Two make sure my animatedImg class is included. Three, make a call to the passing in the array, so this;
Snipt.net is dead - replace code sample from: "http://snipt.net/embed/43547100f4e6b5dc03399183e335d5c2"
Makes this


Animation Type
Notice how ball one appears to be only playing half of the animation while ball two bounces smoothly up and down. The set of images we have passed to our AnimatedImg object is in fact only half a ball bounce. When creating an AnimatedImg you can dictate how it plays from the start. there are 7 types, and they are referenced through a 0 index array. The values are; 0 = "stopped", 1 = "playOnce", 2 = "repeat", 3 = "playBackwardOnce", 4 = "playBackwardRepeat", 5 = "forwardBackwardOnce", 6 = "forwardBackwardRepeat".
Methods and Events
Now for the exciting stuff adding interaction to your AnimatedImg. For this example I dug up an old favorite, Chance the original character from my old Skate-er-Guy game. Click on him and he'll do a hard flip for you. Click on him again and he'll reverse his play direction! Anyways, here is the code used to make the skater interaction.
There is some slight buggyness with mouse based events I'm trying to work out. Currently, the mouse events stand a chance of not registering from what I believe is the click happening as the image is changing frames, thus the AnimatedImg has no clickable area for that brief instance.
Well I'm not into super gigantic posts so I'll cut to the chase, if you would like to use this class for a personal project or to just play with feel free to DOWNLOAD THE EXAMPLE PACKAGE.
JavaScript XML Node Array
7/14/09
To accompany the makeXML function, here is is a method I use to get an array of node values by node name. I do realise I need to put this in a packaged product to help understanding of the js-xml methods. But this should prove helpful to some I hope. GRAB THE VALUE OF SEVERAL NODES!!!
Snipt.net is dead. Replace link to "http://snipt.net/embed/4647be30cc3a6705a36857c7a37e0319"
XML to JavaScript Structure Return
7/12/09
I can't help it, I love xml. For those of use who started out as artists and designers, denied the privilege of touching the database, xml was our bypass. Anyways, this is nothing to special, but it is something very useful. This little function returns the proper xml format of you clients browser to your javascript.
example:
var xmlDoc = makeXML();
xmlDoc.load("some_doc.xml");
Snipt.net is dead. Replace link to "http://snipt.net/embed/737d96d3b7cd393cf4ba9a742b3b3fb8"